Getting Started with Next.js
In this guide, you'll add Beyond Identity passkeys (public-private key pairs) for user authentication to a Next application that uses NextAuth.
This guide uses Visual Studio Code (VS Code) and the example app from NextAuth.js. However, you can use these concepts to add Beyond Identity to any web app using your Beyond Identity tenant and OAuth2/OIDC.
This guide uses the Hosted Web Beyond Identity authenticator type, which has a lighter footprint in your app. For guidance using the Embedded SDK authenticator type, see the NextAuth integration guide. See the Authentication article for more information on authenticator types.
1. Sign up for a developer account​
Go to the sign-up form to get your free Beyond Identity developer account.
After you've completed the form, you won't need to wait for an email. Instead, you'll immediately arrive at the Beyond Identity Admin Console with a passkey and tenant created for you automatically. The Admin Console is where you can configure the example application to authorize and authenticate users with Beyond Identity.
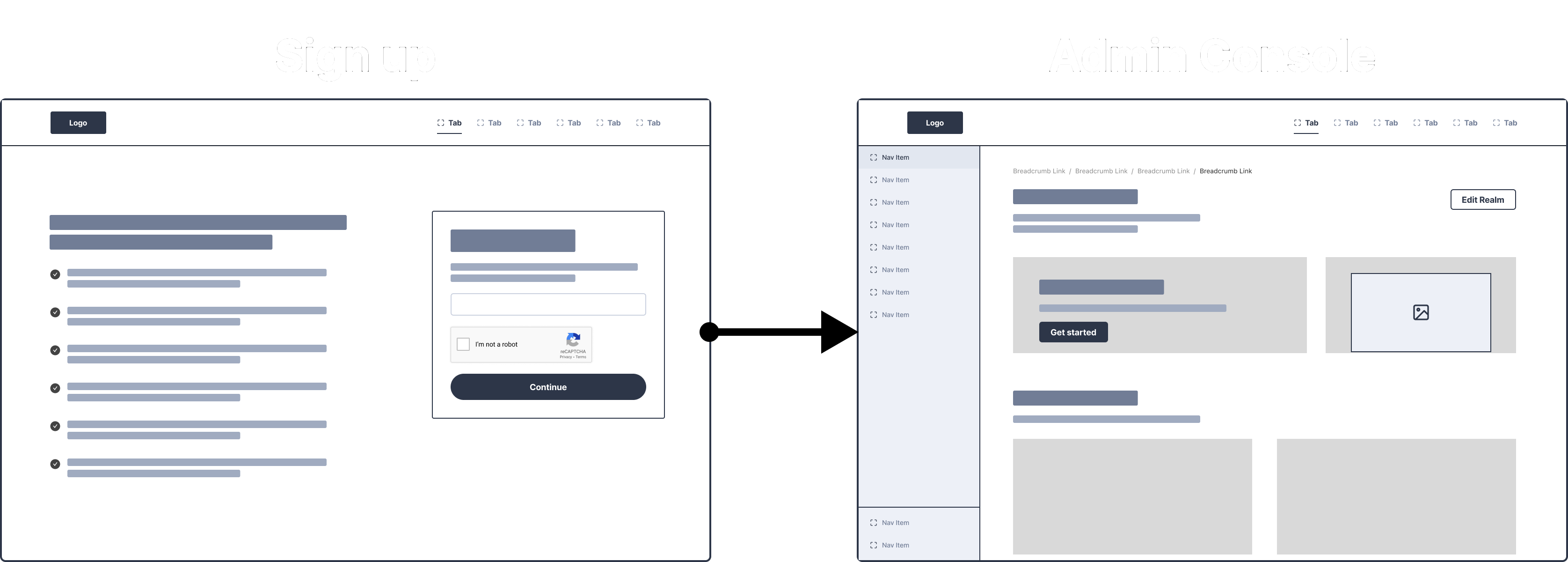
The sign-up process, in a nutshell, automatically creates the following:
A free Beyond Identity developer account in your selected region, which hosts your tenant. Beyond Identity offers US and EU regions that meet regional data requirements and privacy laws.
A tenant with a unique identifier representing the root container for all other cloud components in your configuration. A tenant represents an organization in the Beyond Identity Cloud.
A Beyond Identity Admin Realm, which is created and populated with an admin identity linked to your email. It is the home for your Beyond Identity Admin Console and Beyond Identity Management API, which only supports the client credentials flow.
The Admin Console Access User Group and rule-based authentication policy using the Beyond Identity Admin Console application and this user group.
A Universal Passkey for accessing the Admin Console, bound to your identity and stored on your device or browser. You'll be logged into the Admin Console automatically during this process step. You'll log in without a password when logging into the console from the same device or browser.
A bootstrap Realm and Application called My First Realm and My First Application, to be used with our Getting Started with NextJS guide.
2. Clone the sample app​
Download and install the tools​
First, make sure you've downloaded and installed the following tools on the development machine:
You can verify that node, npm, and git are installed using the following commands:
node -v
npm -v
git --version
Create the project​
On the development machine, create a new folder for your project.
In VS Code, select File > Open Folder and select your project folder.
Open the terminal (View > Terminal) and clone the example app, change to the app's directory, and install the app's dependencies.
git clone https://github.com/nextauthjs/next-auth-example.git
cd next-auth-example
npm install
3. Configure Beyond Identity​
In this step, you'll create a set of Beyond Identity resources containing your end users' identities and the configuration. We've configured these resources already as part of the developer sign up as My First Realm and My First Application so you can go straight to Complete the configuration.
If you are signing in from a different computer or browser, you can enter the email address you used when you signed up. Beyond Identity will send you a one-time link to sign in and enroll a passkey from the new browser.
Create a new realm​
The first step is to create a new realm in the Beyond Identity Admin Console.
We've already created a new application for you called My First Application, so you can skip straight to Complete the configuration below. But if you want to go through the steps of creating a new realm yourself, follow the steps below.
Create a new realm
Creating a realm from the Beyond Identity Admin Console is easy.
In the Admin Console, under Tenant Management, select Go to realm > Create new realm.
Enter a name for your realm and click Create realm.
In the confirmation dialog, switch to the new realm.
Create a hosted web application​
The next step is to create a new Application containing the configuration for your authentication.
We've already created a new application for you called My First Application, so you can skip straight to Complete the configuration below. We will also outline the steps below if you want to go through the process of creating a hosted web application yourself.
Create a hosted web application
From the Admin Console, under Authentication, select Apps > Add new app.
Give your application a name.
On the External Protocol tab, use the following values to complete this tab.
Property Value Display Name Descriptive name you choose Protocol OIDC Why OIDC?
OAuth2 is primarily an authorization framework for resource access, while OIDC builds on OAuth2 to provide an identity layer for authentication, allowing client applications to obtain information about the authenticated user. Both protocols are often used together in modern applications to provide a comprehensive solution for secure authentication and authorization.
Client Type Confidential Why Confidential?
A "confidential" client type is ideal when your application can securely store a client secret and requires enhanced security features for token exchange and accessing user-specific resources. If your application runs in an untrusted environment or you cannot securely manage a client secret, a "public" client type might be more appropriate.
PKCE S256 Why S256?
Choosing the "S256" PKCE type is strongly recommended for public clients like SPAs or mobile apps. It offers a significant security improvement over the "disabled" and "plain" options, effectively protecting against code interception attacks, which are a major concern in less secure client environments. By using "S256" PKCE, you can ensure a higher level of security for your OAuth 2.0 and OIDC flows and better protect your users' data and resources.
Redirect URIs Use your application's App Scheme or Universal URL.
Your redirect URI follows the pattern:http://localhost:3000/api/auth/callback/beyondidentity
Token Endpoint Auth Method Client Secret Basic Why Client Secret Basic?
If your OIDC client is a confidential client and you can securely store the client secret, "client_secret_basic" is the recommended option due to its security benefits and broad support across various authorization servers. However, for public clients or environments where storing the client secret securely is challenging, you might opt for alternative authentication methods like "client_secret_post" or "none," while understanding the trade-offs in security.
Grant Type Authorization Code Why Authorization Code?
The "authorization_code" grant type is suitable for confidential clients, especially when your application needs to access user-specific resources, requires Single Sign-On (SSO) support, and prioritizes security in the authentication process. It provides a secure and standardized way to obtain access to user data and resources without exposing user credentials to the client application.
All other options Use the default values for the remaining options Click the Authenticator Config tab
Property Value Configuration Type Hosted Web Why Hosted Web?
Hosted Web handles passkey registration and authentication for you, including generating new passkeys, presenting users with authenticator choice options as needed, and validating passkey assertions. With this model, your app simply needs to redirect to Beyond Identity's hosted web authenticator, and we do the rest.
Authentication Profile Use the recommended values for the remaining options Click Submit to save the new app.
Complete the configuration​
In this step, you'll collect the configuration elements you must set environment variables in the sample app's .env.config
table.
The table below lists these elements and where to find them in the tenant. If you skipped straight to this step, navigate to Authentication > Apps > My First Application to obtain the variables.
Property Name | Where to find the value |
---|---|
BEYOND_IDENTITY_CLIENT_ID | Copy your app’s Client ID from the External Protocol tab on the app’s configuration page in your tenant |
BEYOND_IDENTITY_CLIENT_SECRET | Copy your app’s Client Secret from the External Protocol tab on the app’s configuration page in your tenant |
BEYOND_IDENTITY_DISCOVERY | Copy your app’s Discovery Endpoint from the External Protocol tab on the app’s configuration page in your tenant |
Guess what? You're halfway there! So far in your getting started journey, you should have completed the following:
Signed up for a free Beyond Identity developer account, which created a passkey, tenant, and Admin Realm for you automatically.
Cloned the example application.
Created (or used the pre-existing) the configuration in your tenant, including a realm and an application.
In the next step, you'll set up the example application locally:
Create and populate .env.local file
Create Beyond Identity as an OAuth provider in the app
Create identities and run the app
Don't forget, if you get stuck, let us know in our Slack community.
4. Configure Beyond Identity as an OAuth provider​
In this step, set your environment variables and configure Beyond Identity as an OAuth provider in the sample app.
Environment variables and .env.local file​
Make the following changes to your NextAuth example app project to set up your environment variables:
Make a copy of the file .env.local.example called .env.local
In .env.local, configure the following variables from your Beyond Identity tenant:
Variable Name Where to find the value NEXTAUTH_URL
Use the default value of http://localhost:3000 NEXTAUTH_SECRET
generate one here: https://generate-secret.vercel.app/32 BEYOND_IDENTITY_CLIENT_ID
Copy your app’s Client ID from the External Protocol tab on the app’s configuration page in your tenant BEYOND_IDENTITY_CLIENT_SECRET
Copy your app’s Client Secret from the External Protocol tab on the app’s configuration page in your tenant BEYOND_IDENTITY_DISCOVERY
Copy your app’s Discovery Endpoint from the External Protocol tab on the app’s configuration page in your tenant Copy and paste the value from Applications > {New Application} > External Protocol > Issuer.
Your .env.local file's contents should look something like the example below:
.env.local# Next Auth
NEXTAUTH_URL=http://localhost:3000
NEXTAUTH_SECRET=df118e1458e3490b22dc8e2d2a6f0247
...
# Beyond Identity
BEYOND_IDENTITY_CLIENT_ID=Njlb6brHbCofRyCWGkiUQweTz
BEYOND_IDENTITY_CLIENT_SECRET=zs2oENddYNes ... 7uamRhvRJ9d3ZJcI97X
BEYOND_IDENTITY_DISCOVERY=https://auth-us.beyondidentity.com/v1/tenants/000111222333/realms/444555666777888/applications/1233449b-11c4-4884-b622-0bfbaefd44d3Update the contents of the file process.d.ts to the following:
declare namespace NodeJS {
export interface ProcessEnv {
NEXTAUTH_URL: string
NEXTAUTH_SECRET: string
BEYOND_IDENTITY_CLIENT_ID: string
BEYOND_IDENTITY_CLIENT_SECRET: string
BEYOND_IDENTITY_DISCOVERY: string
}
}
Create OAuth provider​
Make the following change to your NextAuth example app project to configure Beyond Identity as an OAuth provider:
Next.js Version | Route |
---|---|
12 | Create a route at pages/api/auth/[...nextauth].js. |
13 | Create a route at app/api/auth/[...nextauth]/route.ts. |
Inside the providers
array, add the following entry:
{
id: "beyondidentity",
name: "Beyond Identity",
type: "oauth",
wellKnown: process.env.BEYOND_IDENTITY_DISCOVERY,
authorization: { params: { scope: "openid" } },
clientId: process.env.BEYOND_IDENTITY_CLIENT_ID,
clientSecret: process.env.BEYOND_IDENTITY_CLIENT_SECRET,
idToken: true,
checks: ["pkce", "state"],
profile(profile) {
return {
id: profile.sub,
name: profile.sub,
email: profile.sub,
}
},
},
Double-check the package.json file​
Inside the package.json file, ensure that the scripts that run the app use the correct port for the following URLs:
- your NEXTAUTH_URL, such as 'http://localhost:3000'
- the app's configured redirect URI in the BI tenant, such as 'http://localhost:3000/api/auth/callback/beyondidentity'
Next's default port is 3000, so you do not need to specify a port if you use port 3000.
If you are using a different port in your NEXTAUTH_URL, for example, '8083', the app's redirect URI would be http://localhost:8083/api/auth/callback/beyondidentity, and the script should start the app using the -p option, for example "next -p 8083"
Next, you'll create users and passkeys test the app.
5. Create users and passkeys​
You are ready to test without writing more code because you created an app with the 'Hosted Web' authentication configuration type. The 'Hosted Web' authentication configuration handles user registration and authentication. If you want more customization, consider using the 'Embedded SDK' configuration type. For more information, see the NextAuth integration guide.
6. Run the example application and test sign in​
In this step, you'll start the backend and frontend to run the example app locally.
In VS Code, run:
npm run dev
From the browser where you created the passkey above, navigate to
http://localhost:3000
.Sign in to the app, as shown below.
Registration and passkey binding happens in the Hosted Web UI. The user gets prompted to provide an email for verification on the first authentication. They'll have the option to generate a passkey on trusted devices, which they'll use on this device each time they authenticate.